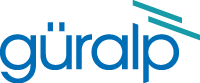
Chapter 4. Appendices
4.1 Operators
The available operators (Op) are given in the following table, along with their priorities (Pri) and syntax. Unlimited levels of parentheses (priority 0, which has highest precedence) can be used for grouping of sub-expressions.
Op | Pri | Syntax | Description |
= | 4 | {boolean} = {value} = {value} | Equality test |
<> | 4 | {boolean} = {value} <> {value} | Inequality test |
>= | 4 | {boolean} = {value} >= {value} | Greater than or equal to test |
<= | 4 | {boolean} = {value} <= {value} | Less than or equal to test |
> | 4 | {boolean} = {value} > {value} | Greater than test |
< | 4 | {boolean} = {value} < {value} | Less than test |
+ | 3 | {numeric} = {numeric} + {numeric} | Numeric summation |
- | 3 | {numeric} = {numeric} - {numeric} | Numeric subtraction |
Or | 3 | {boolean} = {boolean} Or {boolean} | Boolean OR test |
Xor | 3 | {boolean} = {boolean} Xor {boolean} | Boolean exclusive OR test |
* | 2 | {numeric} = {numeric} * {numeric} | Numeric multiplication |
/ | 2 | {numeric} = {numeric} / {numeric} | Numeric division |
^ | 2 | {numeric} = {numeric} ^ {numeric} | Numeric power |
Div | 2 | {integer} = {numeric} Div {numeric} | Numeric integer division |
Mod | 2 | {numeric} = {numeric} Mod {numeric} | Numeric modula division |
And | 2 | {boolean} = {boolean} And {boolean} | Boolean AND test |
SHR | 2 | {numeric} = {numeric} SHR {numeric} | Bit-wise shift right |
SHL | 2 | {numeric} = {numeric} SHL {numeric} | Bit-wise shift left |
Not | 1 | {boolean} = Not {boolean} | Boolean negation |
- | 1 | {numeric} = - {numeric} | Numeric negation |
( ) | 0 | ( {expression} ) | Sub expression grouping |
4.2 Functions
Not all of the functions in this section have an obvious use in this context. They are included for completeness but they may not have been tested. Please satisfy yourself about suitability for purpose before deploying any of these in a production environment.
4.2.1 Numeric functions
Some of the functions below may return real (or even complex) values but GCF can only encode integer values. The last 5 results for each expression at kept in memory as full double-precision reals in order to allow for the creation of recursive functions (e.g. IIR filters) with maximum precision.
Otherwise, if an expression produces real values as a result, they will be "clipped" to lie between ±231 and converted to integers before being fed into the GCF compressor. The behaviour with complex results is undefined.
Symbol | Syntax |
MinInteger | {integer} = MinInteger |
MaxInteger | {integer} = MaxInteger |
AsString | {string} = AsString( {value} ) |
AsInteger | {integer} = AsInteger( {value} ) |
AsReal | {real} = AsReal( {value} ) |
Max | {value} = Max( {value}, [...] ) |
Min | {value} = Min( {value}, [...] ) |
Average | {numeric} = Average( {numeric}, [...] ) |
Ranged | {value} = Ranged( {minvalue}, {maxvalue}, {value} ) |
Round | {numeric} = Round( {numeric} ) |
Trunc | {integer} = Trunc( {numeric} ) |
Absolute | {numeric} = Absolute( {numeric} ) |
Sign | {integer} = Sign( {numeric} ) |
Square | {numeric} = Square( {numeric} ) |
SquareRoot | {numeric} = SquareRoot( {numeric} ) |
RandomRange | {numeric} = RandomRange( {minvalue}, {maxvalue} ) |
RandomFrom | {value} = RandomFrom( {value}, [...] ) |
Random | {numeric} = Random |
IfThen | {value} = IfThen( {boolean}, {trueval}, {falseval} ) |
MinReal | {numeric} = MinReal |
MaxReal | {numeric} = MaxReal |
Pi | {numeric} = Pi |
Exp | {numeric} = Exp( {numeric} ) |
Numerator | {numeric} = Numerator( {numeric} ) |
Denominator | {numeric} = Denominator( {numeric} ) |
Ceil | {numeric} = Ceil( {numeric} ) |
Floor | {numeric} = Floor( {numeric} ) |
Tan | {numeric} = Tan( {numeric} ) |
Sin | {numeric} = Sin( {numeric} ) |
Cos | {numeric} = Cos( {numeric} ) |
ArcTan | {numeric} = ArcTan( {numeric} ) |
ArcSin | {numeric} = ArcSin( {numeric} ) |
ArcCos | {numeric} = ArcCos( {numeric} ) |
TanH | {numeric} = TanH( {numeric} ) |
SinH | {numeric} = SinH( {numeric} ) |
CosH | {numeric} = CosH( {numeric} ) |
ArcTanH | {numeric} = ArcTanH( {numeric} ) |
ArcSinH | {numeric} = ArcSinH( {numeric} ) |
ArcCosH | {numeric} = ArcCosH( {numeric} ) |
RadToGrad | {numeric} = RadToGrad( {numeric} ) |
RadToDeg | {numeric} = RadToDeg( {numeric} ) |
RadToCycle | {numeric} = RadToCycle( {numeric} ) |
GradToRad | {numeric} = GradToRad( {numeric} ) |
DegToRad | {numeric} = DegToRad( {numeric} ) |
CycleToRad | {numeric} = CycleToRad( {numeric} ) |
Log10 | {numeric} = Log10( {numeric} ) |
Log2 | {numeric} = Log2( {numeric} ) |
LogN | {numeric} = LogN( {base}, {numeric} ) |
Ln | {numeric} = Ln( {numeric} ) |
GradToDeg | {numeric} = GradToDeg( {numeric} ) |
GradToCycle | {numeric} = GradToCycle( {numeric} ) |
DegToGrad | {numeric} = DegToGrad( {numeric} ) |
DegToCycle | {numeric} = DegToCycle( {numeric} ) |
CycleToGrad | {numeric} = CycleToGrad( {numeric} ) |
CycleToDeg | {numeric} = CycleToDeg( {numeric} ) |
CoTan | {numeric} = CoTan( {numeric} ) |
ArcTan2 | {numeric} = ArcTan2( {xnumeric}, {ynumeric} ) |
Hypot | {numeric} = Hypot( {xnumeric}, {ynumeric} ) |
LnXP1 | {numeric} = LnXP1( {numeric} ) |
RandG | {numeric} = RandG( {mean}, {stddev} ) |
LdExp | {numeric} = LdExp( {numeric}, {power} ) |
Mean | {numeric} = Mean( {numeric}, [...] ) |
Sum | {numeric} = Sum( {numeric}, [...] ) |
SumOfSquares | {numeric} = SumOfSquares( {numeric}, [...] ) |
4.2.2 Boolean functions
These functions can be used in conjunction with IfThen() to select between values.
Symbol | Syntax |
True | {boolean} = True |
False | {boolean} = False |
AsBoolean | {boolean} = AsBoolean( {value} ) |
IsEmpty | {boolean} = IsEmpty( {value} ) |
IsNull | {boolean} = IsNull( {value} ) |
InRange | {boolean} = InRange( {minvalue}, {maxvalue}, {value} ) |
4.2.3 Dates and datetimes
Symbol | Syntax |
Now | {datetime} = Now |
TheDate | {datetime} = TheDate |
TheTime | {datetime} = TheTime |
AsDateTime | {datetime} = AsDateTime( {value} ) |
DateOf | {date} = DateOf( {datetime} ) |
TimeOf | {time} = TimeOf( {datetime} ) |
YearOf | {numeric} = YearOf( {datetime} ) |
MonthOf | {numeric} = MonthOf( {datetime} ) |
WeekOf | {numeric} = WeekOf( {datetime} ) |
DayOf | {numeric} = DayOf( {datetime} ) |
HourOf | {numeric} = HourOf( {datetime} ) |
MinuteOf | {numeric} = MinuteOf( {datetime} ) |
SecondOf | {numeric} = SecondOf( {datetime} ) |
MilliSecondOf | {numeric} = MilliSecondOf( {datetime} ) |
DayOfYearOf | {numeric} = DayOfYearOf( {datetime} ) |
DayOfWeekOf | {numeric} = DayOfWeekOf( {datetime} ) |
DateFor | {datetime} = DateFor( {year}, {month}, {day} ) |
TimeFor | {datetime} = TimeFor( {hour}, {minute}, {seconds}, {milliseconds} ) |
DateTimeFor | {datetime} = DateTimeFor( {year}, {month}, {day}, {hour}, {minute}, {seconds}, {milliseconds} ) |
4.2.4 Extended dates and datetimes
Symbol | Syntax |
IsLeapYear | {boolean} = IsLeapYear( {year} ) |
IsInLeapYear | {boolean} = IsInLeapYear( {datetime} ) |
DaysInAMonth | {integer} = DaysInAMonth( {year}, {month} ) |
DaysInMonth | {integer} = DaysInMonth( {datetime} ) |
DaysInAYear | {integer} = DaysInAYear( {year} ) |
DaysInYear | {integer} = DaysInYear( {datetime} ) |
StartOfYear | {datetime} = StartOfYear( {datetime} ) |
EndOfYear | {datetime} = EndOfYear( {datetime} ) |
StartOfMonth | {datetime} = StartOfMonth( {datetime} ) |
EndOfMonth | {datetime} = EndOfMonth( {datetime} ) |
StartOfWeek | {datetime} = StartOfWeek( {datetime} ) |
EndOfWeek | {datetime} = EndOfWeek( {datetime} ) |
StartOfDay | {datetime} = StartOfDay( {datetime} ) |
EndOfDay | {datetime} = EndOfDay( {datetime} ) |
MonthOfYear | {numeric} = MonthOfYear( {datetime} ) |
WeekOfYear | {numeric} = WeekOfYear( {datetime} ) |
DayOfYear | {numeric} = DayOfYear( {datetime} ) |
HourOfYear | {numeric} = HourOfYear( {datetime} ) |
MinuteOfYear | {numeric} = MinuteOfYear( {datetime} ) |
SecondOfYear | {numeric} = SecondOfYear( {datetime} ) |
MilliSecondOfYear | {numeric} = MilliSecondOfYear( {datetime} ) |
WeekOfMonth | {numeric} = WeekOfMonth( {datetime} ) |
DayOfMonth | {numeric} = DayOfMonth( {datetime} ) |
HourOfMonth | {numeric} = HourOfMonth( {datetime} ) |
SecondOfMonth | {numeric} = SecondOfMonth( {datetime} ) |
MilliSecondOfMonth | {numeric} = MilliSecondOfMonth( {datetime} ) |
DayOfWeek | {numeric} = DayOfWeek( {datetime} ) |
HourOfWeek | {numeric} = HourOfWeek( {datetime} ) |
MinuteOfWeek | {numeric} = MinuteOfWeek( {datetime} ) |
SecondOfWeek | {numeric} = SecondOfWeek( {datetime} ) |
MilliSecondOfWeek | {numeric} = MilliSecondOfWeek( {datetime} ) |
HourOfDay | {numeric} = HourOfDay( {datetime} ) |
MinuteOfDay | {numeric} = MinuteOfDay( {datetime} ) |
SecondOfDay | {numeric} = SecondOfDay( {datetime} ) |
MilliSecondOfDay | {numeric} = MilliSecondOfDay( {datetime} ) |
MinuteOfHour | {numeric} = MinuteOfHour( {datetime} ) |
SecondOfHour | {numeric} = SecondOfHour( {datetime} ) |
MilliSecondOfHour | {numeric} = MilliSecondOfHour( {datetime} ) |
SecondOfMinute | {numeric} = SecondOfMinute( {datetime} ) |
MilliSecondOfMinute | {numeric} = MilliSecondOfMinute( {datetime} ) |
MilliSecondOfSecond | {numeric} = MilliSecondOfSecond( {datetime} ) |
4.2.5 Time-spans
Symbol | Syntax |
WithinPastYears | {boolean} = WithinPastYears( {now}, {then}, {years} ) |
WithinPastMonths | {boolean} = WithinPastMonths( {now}, {then}, {months} ) |
WithinPastWeeks | {boolean} = WithinPastWeeks( {now}, {then}, {weeks} ) |
WithinPastDays | {boolean} = WithinPastDays( {now}, {then}, {days} ) |
WithinPastHours | {boolean} = WithinPastHours( {now}, {then}, {hours} ) |
WithinPastMinutes | {boolean} = WithinPastMinutes( {now}, {then}, {minutes} ) |
WithinPastSeconds | {boolean} = WithinPastSeconds( {now}, {then}, {seconds} ) |
WithinPastMilliSeconds | {boolean} = WithinPastMilliSeconds( {now}, {then}, {milliseconds} ) |
YearsBetween | {numeric} = YearsBetween( {now}, {then} ) |
MonthsBetween | {numeric} = MonthsBetween( {now}, {then} ) |
WeeksBetween | {numeric} = WeeksBetween( {now}, {then} ) |
DaysBetween | {numeric} = DaysBetween( {now}, {then} ) |
HoursBetween | {numeric} = HoursBetween( {now}, {then} ) |
MinutesBetween | {numeric} = MinutesBetween( {now}, {then} ) |
SecondsBetween | {numeric} = SecondsBetween( {now}, {then} ) |
MilliSecondsBetween | {numeric} = MilliSecondsBetween( {now}, {then} ) |
YearSpan | {numeric} = YearSpan( {now}, {then} ) |
MonthSpan | {numeric} = MonthSpan( {now}, {then} ) |
WeekSpan | {numeric} = WeekSpan( {now}, {then} ) |
DaySpan | {numeric} = DaySpan( {now}, {then} ) |
HourSpan | {numeric} = HourSpan( {now}, {then} ) |
MinuteSpan | {numeric} = MinuteSpan( {now}, {then} ) |
SecondSpan | {numeric} = SecondSpan( {now}, {then} ) |
MilliSecondSpan | {numeric} = MilliSecondSpan( {now}, {then} ) |
4.2.6 Complex-valued functions
Complex values may be used only as interim results in expressions.
Symbol | Syntax |
AsComplex | {complex} = AsComplex( {value} ) |
IsComplex | {boolean} = IsComplex( {value} ) |
RealOf | {numeric} = RealOf( {value} ) |
ImaginaryOf | {numeric} = ImaginaryOf( {value} ) |
ComplexFor | {complex} = ComplexFor( {real}, {imaginary} ) |
Simplify | {value} = Simplify( {value} ) |
Conjugate | {value} = Conjugate( {value} ) |
Inverse | {value} = Inverse( {value} ) |
TimesPosI | {value} = TimesPosI( {value} ) |
TimesNegI | {value} = TimesNegI( {value} ) |
Norm | {value} = Norm( {value} ) |
Angle | {value} = Angle( {value} ) |
Exp | {value} = Exp( {value} ) |
Ln | {value} = Ln( {value} ) |
SquareRoot | {value} = SquareRoot( {value} ) |
Cos | {value} = Cos( {value} ) |
Sin | {value} = Sin( {value} ) |
Tan | {value} = Tan( {value} ) |
ArcCos | {value} = ArcCos( {value} ) |
ArcSin | {value} = ArcSin( {value} ) |
ArcTan | {value} = ArcTan( {value} ) |
CosH | {value} = CosH( {value} ) |
SinH | {value} = SinH( {value} ) |
TanH | {value} = TanH( {value} ) |
ArcCosH | {value} = ArcCosH( {value} ) |
ArcSinH | {value} = ArcSinH( {value} ) |
ArcTanH | {value} = ArcTanH( {value} ) |